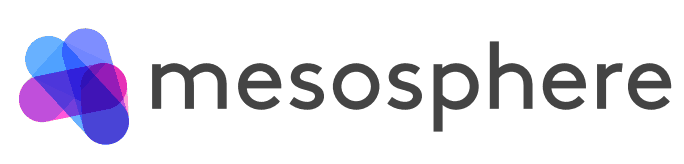
Installation and setup of Mesos using Mesosphere
Last updated: 10-Oct-2015
In this section I will explain how to setup a very basic Mesos cluster. To keep things as clear as possible I eliminated most of the fancy stuff. Later on I will discuss more advances examples. You can download all the code directly from GitHub with the button below.
Download
Note: many thanks to DigitalOcean.com. I used their explanation as an important reference for this article.
Configuration of a Mesos Master
In this first example we will use only one Master. First we create the VM where the Master will run on. Mesos runs on Linux (64 Bit) and Mac OS X (64 Bit). For this example I will use Ubuntu, and take a standard Vagrant box called ubuntu/trusty64 as a basis. The Vagrantfile is pretty straightforward. We assign a fixed ip address, to make configuration easy. You can change this in any ip address that you personally prefer. After creation of the VM a Bash script called bootmaster1.sh is run.Note: Vagrant runs this script under root, which is why I don’t use sudo in this simple example.
# -*- mode: ruby -*-
# vi: set ft=ruby :
Vagrant.configure(2) do |config|
config.vm.box = "ubuntu/trusty64"
config.vm.network "private_network", ip: "192.168.33.46"
config.vm.provider "virtualbox" do |vb|
vb.memory = 2048
vb.name = "master1c"
end
config.vm.provision :shell, :path => "scripts/bootmaster1.sh"
end
The Bash script will take care of all the configuration. First you have to add the Mesosphere Repositories to your Host. The standard way of doing this is as follows:
apt-key adv --keyserver keyserver.ubuntu.com --recv E56151BF
DISTRO=$(lsb_release -is | tr '[:upper:]' '[:lower:]')
CODENAME=$(lsb_release -cs)
echo "deb http://repos.mesosphere.io/${DISTRO} ${CODENAME} main" | \
sudo tee /etc/apt/sources.list.d/mesosphere.list
Now you have to update the system to make the Mesosphere libraries available. After that is done you can install all components with one simple command. All components means: Mesos, Marathon, Chronos and Zookeeper.
apt-get -y update
apt-get install -y mesosphere
Ok, all components have been installed, but we still need to configure them.
We will start with the configuration of Zookeeper.
Zookeeper needs to know which machines will be part of the Zookeeper cluster. In our case this cluster consists of all the Mesos Masters. Note: In this first example we have only one master, but if you have more you have to repeat this process on every master node.
Step 1:
Specify the ip addresses of all the Mesos Masters in the file /etc/mesos/zk. This file contains one line with the ip addresses of the masters connected to the zookeeper port (2181).
Step 2:
Identify every master with a unique ID number in the range of 1-255. It is not mandatory, but the logical choice is to assign the first master with 1, the second with 2, etc. This ID must be set in the file /etc/zookeeper/conf/myid.
Step 3:
Map the ID's of the masters to the corresponding ip addresses. This is done by adding one line per master node in the file /etc/zookeeper/conf/zoo.cfg. Two additional ports must be specified here for Zookeeper to function correctly. Port 2888 is for communication with the leader. Post 3888 is used to elect a leader.
In our script the configuration for this first example looks like this:
echo "zk://192.168.33.46:2181/mesos" > /etc/mesos/zk
echo "1" > /etc/zookeeper/conf/myid
echo "server.1=192.168.33.46:2888:3888" >> /etc/zookeeper/conf/zoo.cfg
Configuration of Mesos
Step 1:
Specify the number of Mesos Masters that must be available for the Zookeeper cluster to function. This is called the Quorum of the Zookeeper cluster. Since we only have one master in our first example this value is set to 1. The file where this value is set is /etc/mesos-master/quorum.
Step 2:
Specify the ip address of the master server. This is done in the file /etc/mesos-master/ip
Step 3:
Specify the hostname of the master server. This is done in the file /etc/mesos-master/hostname. For the sake of simplicity we use the ip address of the master server as the hostname.
The code in our script will look something like this:
echo "1" > /etc/mesos-master/quorum
echo "192.168.33.46" > /etc/mesos-master/ip
echo "192.168.33.46" > /etc/mesos-master/hostname
Configuration of Marathon
Step 1:
Create the configuration directory /etc/marathon/conf for Marathon. Normally this is not done automatically, so we have to do it in the script.
Step 2:
Specify the hostname of the master server in the file /etc/marathon/conf/hostname. We will use the ip address of the server for this.
Step 3:
Connect Marathon to the Zookeeper cluster. For Marathon the same principle applies as for Mesos. Only one marathon instance will be active. If you connect to any of the other instances, Zookeeper will automatically route you to the master server. To connect marathon to the Zookeeper cluster you specify all zookeeper masters in the file /etc/marathon/conf/master.
Step 4:
Allow Marathon to store its state information in the Zookeeper cluster. With this last step we will make sure we are routed to the active master, and that other masters can take over the running activities in case of a failure of the leading master. To do this add the list of masters to the file /etc/marathon/conf/zk.
The code in our script will be:
mkdir -p /etc/marathon/conf
echo "192.168.33.46" > /etc/marathon/conf/hostname
echo "zk://192.168.33.46:2181/mesos" > /etc/marathon/conf/master
echo "zk://192.168.33.46:2181/marathon" > /etc/marathon/conf/zk
Ok, everything is configured. Now we need to restart the services the activate our settings.
restart zookeeper
start mesos-master
start marathon
As an optional step you can disable the Mesos Slave on the Mesos Master server.
stop mesos-slave
echo manual | tee /etc/init/mesos-slave.override
To see if it works you can access the webinterfaces of Mesos and Marathon.
Mesos: http://192.168.33.46:5050
Marathon: http://192.168.33.46:8080
Configuration of a Mesos Slave
First we need to create a Virtual Machine for the Mesos Slave. This VM will connect to the Mesos Master we already configured. The Vagrantfile for this example is very simple. Note: In the code you can download from GitHub I connect three slaves to the master. Simply repeat the following process for all of the slaves to do this.
# -*- mode: ruby -*-
# vi: set ft=ruby :
Vagrant.configure(2) do |config|
config.vm.box = "ubuntu/trusty64"
config.vm.network "private_network", ip: "192.168.33.51"
config.vm.provider "virtualbox" do |vb|
vb.memory = 2048
vb.name = "slave1"
end
config.vm.provision :shell, :path => "scripts/bootslave1.sh"
end
The script that is run to configure the Mesos Slave is pretty simple as well. First you have to add the Mesosphere Repositories to your Host. This is exactly the same as on the Mesos master, so I will not repeat the code here. The installation itself is a bit different.
apt-get -y update
apt-get install -y mesos
We need to tell the slave who the master(s) are. You can specify this in the file /etc/mesos/zk. As you can see the configuration for this is exactly the same as on the master.
echo "zk://192.168.33.46:2181/mesos" > /etc/mesos/zk
Next we need to specify the ip address and the hostname of the slave. Again we use the ip address as the hostname.
echo "192.168.33.51" > /etc/mesos-slave/ip
echo "192.168.33.51" > /etc/mesos-slave/hostname
To be on the safe side we want to be absolutely sure the services for Zookeeper and the Mesos Master do not run on the Mesos Slave.
stop zookeeper
echo manual | tee /etc/init/zookeeper.override
stop mesos-master
echo manual | tee /etc/init/mesos-master.override
The last step is to start the Mesos Slave.
start mesos-slave
If you go back to the Mesos web interface you should see that a slave has been activated.
If that worked your Mesos cluster is ready for use. In the next section I will discuss how you can run applications in your Mesos cluster.